Nextjs+I18n best practices voor meertalige internationalisering (zoekmachinevriendelijk)
注:这个最佳实践是基于next的pages路由。并不适合app路由。 目录 基本思路 使用next-i18ne […]
注:这个最佳实践是基于next的pages路由。并不适合app路由。
目录
基本思路
使用next-i18next。
在“pages”目录中增加[locales]目录,将主要业务逻辑放到这个目录中。
pages目录中的index等页面用于判断语言,然后调用相应本地化页面逻辑
public目录中增加locales目录,用于翻译文件。
安装
配置
文件目录
.
next.config.js
();
next-i18next.config.js
, , ,, : , : , : , : , : , : , : , : },
],
},
}
对于locales的配置,考虑到对用户展示语言选择的场景,我没有按照网上大多数的配置方式。
lib/getStatic.js
;
;
= (..(() => ({
: {
,
},
}));
= () => ({
: ,
: (),
});
]) => {
?..;
=
() =>
:
这是用来获取语言翻译文件和在服务端翻译的逻辑。
_app.js
;
{ } ;
= () => <> < {} /> </>;
();
注意:如果不使用<Layout>可以直接将这个标签去掉。
_docoument.js
;
, {
,
,
,
,
}
{
() {
.....;
. < =>
<>
< = />
< = = />
</>
<>
< />
< />
</>
</>;
}
}
index.js
;
;
[locales]文件夹
用来实现类似www.xxx.com/es的国际化路由
public/locales文件夹
用来存放翻译文件。
组件
语言选择器:LangSwitcher.js
code
;
..)
= () => {
();
=()=> {
.
.).( => {
(`[]`(`[]`.) {
'/'.'/'
} ``
}
} {
? `/`(`/`) < 0`/`
}
}
= () => {
(
<>< = =>< = ></>< = =></>)
})}
</>
</>
)
}
() {
..( => {
.
使用方法:
引入组件,直接使用<LangSwitcher>,并且lang参数设置为当前的语言。
();
< =></>
本地化Link组件:LocLink.js
;
;
;
= () => {
();
. || ;
.;
.() === 0;
..)
...(. (
<>
< = >
< {}></>
</>
</>
);
};
;
本地化Markdown文件获取和渲染组件:MdRenderer.js
;
() {
();
()
: { : 0 }
})
.( =>())
.( => { (=>{. (
< =><></>)}
</>)
}
使用方法
t函数
- 引入“useTranslation”
- 定义t
- 使用{t(‘xxx’)},即可
;
([]);
{()}
如何获取当前的语言
;
();
.)
如何循环获取语言列表
获取语言列表,可以自定义语言导航。
..( => {
.) (< = = =></>)
(< = = =></>)
})}
内容段落的翻译
如果内容是从后端获取的,那么,这个就不需要了。
但,如果内容存在前端,存在json里非常麻烦,所以,可以存在markdown中,然后在需要展示这个内容的地方获取相应语言版本的markdown文件,然后解析展示出来。
<+ } />
总结
多语言,可以让一个网站获取更多的流量。这个nextjs的多语言最佳实践,充分考虑了SEO的需要。搜索引擎友好。
适合做工具站、关键词站、新闻和内容站。
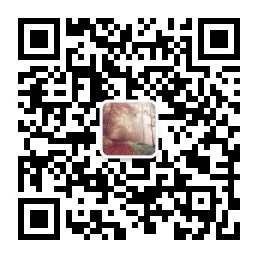
关注我的微信公众号